【コードあり】抽象ファクトリーパターンのC++版サンプルコード
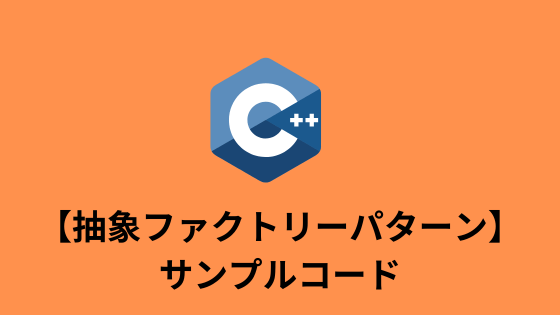
今回はデザインパターンの抽象ファクトリーパターンのサンプルコードを作成しました。
記事の作成に当たってインプットには以下の書籍やサイトを使って勉強しました。
間違い等あればコメントしてもらえると対応します。
目次
抽象ファクトリーパターンの概要
抽象ファクトリーパターンは、関連する一連のオブジェクトを生成するためのクラス階層をカプセル化するデザインパターンです。これにより、特定の具体クラスのインスタンスを直接作成するのではなく、ファクトリークラスを介してオブジェクトの生成を行います。
構造はrefactoring.guruの図がわかりやすいのでそれ見て下さい。
c++のサンプルコード
MacとWindowsのGUIを元にサンプルを作成してみました。
抽象製品クラス(Button.h)
ボタンの抽象クラス。具象クラスで使用するメソッドを宣言します。
#pragma once
#include <iostream>
// 抽象製品
class Shape {
public:
virtual void draw() = 0;
};
抽象製品クラス(TextBox.h)
テキストボックスの抽象クラス
#pragma once
#include <iostream>
// 抽象製品クラス: テキストボックス
class TextBox {
public:
virtual void render() = 0;
};
具象製品クラス(MacButton.h)
Macのボタンクラス。抽象製品クラスで宣言したメソッドを定義します
#include "Button.h"
// 具体製品クラス: Macボタン
class MacButton : public Button {
public:
void render() override {
std::cout << "Render Mac button" << std::endl;
}
};
具象製品クラス(WindowsButton.h)
Windowsのボタンクラス。
#include "Button.h"
// 具体製品クラス: Windowsボタン
class WindowsButton : public Button {
public:
void render() override {
std::cout << "Render Windows button" << std::endl;
}
};
具象製品クラス(MacTextBox.h)
Macのテキストボックスクラス。
#include "TextBox.h"
// 具体製品クラス: Macテキストボックス
class MacTextBox : public TextBox {
public:
void render() override {
std::cout << "Render Mac text box" << std::endl;
}
};
具象製品クラス(WindowsTextBox.h)
WindowsのTextBoxクラス。
#include "TextBox.h"
// 具体製品クラス: Windowsテキストボックス
class WindowsTextBox : public TextBox {
public:
void render() override {
std::cout << "Render Windows text box" << std::endl;
}
};
抽象ファクトリークラス(GUIFactory.h)
抽象ファクトリーでは抽象製品クラス型を返すメソッドを宣言します。
#pragma once
#include <memory>
#include "Button.h"
#include "TextBox.h"
// 抽象ファクトリークラス
class GUIFactory {
public:
virtual std::unique_ptr<Button> createButton() = 0;
virtual std::unique_ptr<TextBox> createTextBox() = 0;
};
具象ファクトリークラス(MacGUIFactory.h)
具象ファクトリークラスは、抽象ファクトリークラスを継承し、対応する具象製品クラスを返すメソッドを定義します。
#include "GUIFactory.h"
#include "MacButton.h"
#include "MacTextBox.h"
// 具体ファクトリークラス: Mac GUIファクトリー
class MacGUIFactory : public GUIFactory {
public:
std::unique_ptr<Button> createButton() override {
return std::make_unique<MacButton>();
}
std::unique_ptr<TextBox> createTextBox() override {
return std::make_unique<MacTextBox>();
}
};
具象ファクトリークラス(WindowsGUIFactory.h)
#include "GUIFactory.h"
#include "WindowsButton.h"
#include "WindowsTestBox.h"
// 具体ファクトリークラス: Windows GUIファクトリー
class WindowsGUIFactory : public GUIFactory {
public:
std::unique_ptr<Button> createButton() override {
return std::make_unique<WindowsButton>();
}
std::unique_ptr<TextBox> createTextBox() override {
return std::make_unique<WindowsTextBox>();
}
};
Main関数(main.cpp)
具象ファクトリーを宣言し、そのメソッドを使用して製品オブジェクトを生成します。製品オブジェクトは抽象クラス型で宣言します。
#include "MacGUIFactory.h"
#include "WindowsGUIFactory.h"
// クライアントコード
int main() {
// Mac GUIファクトリーを使用してボタンとテキストボックスを生成
std::unique_ptr<GUIFactory> macFactory = std::make_unique<MacGUIFactory>();
std::unique_ptr<Button> macButton = macFactory->createButton();
std::unique_ptr<TextBox> macTextBox = macFactory->createTextBox();
macButton->render();
macTextBox->render();
// Windows GUIファクトリーを使用してボタンとテキストボックスを生成
std::unique_ptr<GUIFactory> windowsFactory = std::make_unique<WindowsGUIFactory>();
std::unique_ptr<Button> windowsButton = windowsFactory->createButton();
std::unique_ptr<TextBox> windowsTextBox = windowsFactory->createTextBox();
windowsButton->render();
windowsTextBox->render();
return 0;
}
サンプルコードの実行結果
実行結果は以下のようになります。
Render Mac button
Render Mac text box
Render Windows button
Render Windows text box